목록전체 글 (246)
without haste but without rest
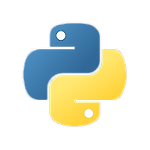
import random def make_score(a=30, b=99, dup=True): names = [chr(name) for name in range(ord('a'), ord('z'))] random.shuffle(names) if dup == True: scores = [[random.randint(a, b) for _ in range(5)] for _ in range(8)] res = list(zip(names, scores)) return res else: scores = [random.sample(range(10, 100), 5) for _ in range(8)] res = list(zip(names, scores)) return res def print_list(score_list, n..

0. 개요 -박스 플롯 - 분산 확인 -바이올린 플롯 - 분산 확인 + 분포 확인 -스캐터 플롯 - 변수들 간의 상관관계 -페어 플롯 - 변수들 간의 상관관계 -히트맵 - 변수들 간의 상관관계 -조인트 플롯 - 스캐터 + 러그 -스왐 플롯 - 분류 문제 -스트립 플롯 - 분류 문제 1. 데이터 로드 """ Exploring """ import pandas as pd # load iris iris = pd.read_csv("iris.csv") iris.head() print(iris.columns) print(iris) 컬럼 네임들이 짤려서 나온다. # 컬럼 이름 변경하기 iris.rename(columns = {iris.columns[0] : 'Sepal.Length', iris.columns[1] : ..

import random # 0.0 ~ 1.0 미만 실수 반환 random.random() # a 이상 b 미만 정수 반환 random.randint(a, b) # a 이상 b 미만 정수 범위에서 interval 배수 반환 random.randrange(a, b, interval) # a 이상 b 미만 숫자 중 n개를 중복없이 반환 random.sample(range(10, 100), n)) sample_list = [1, 2, 3, 4, 5] # 랜덤 선택 # 매개변수로 받은 리스트에서 인자 n개 추출 # default 개수는 1개 random.choice(sample_list, n) # 리스트 셔플 random.shuffle(sample_list)

/* title - 교재 뱅킹 시뮬레이션 코드 수정 원형큐를 이용하여 뱅킹 업무처리 시스템 구현 */ #include #include #include #define MAX_QUEUE_SIZE 20 // ================ 원형큐 정의부 시작 ================= typedef struct { // 요소 타입 int id; int arrival_time; int service_time; } element;// 교체! // ================ 원형큐 정의부 종료 ================= // ===== 원형큐 코드 시작 ====== typedef struct { // 큐 타입 element data[MAX_QUEUE_SIZE]; int front, rear; } Que..

# include # include // 프로그램 5.2에서 다음과 같은 부분을 복사한다. // ================ 원형큐 정의부 시작 ================= typedef struct { // 요소 타입 int id; int arrival_time; int service_time; int start_time; } element;// 교체! // ================ 원형큐 정의부 종료 ================= // ===== 원형큐 코드 시작 ====== #define MAX_QUEUE_SIZE 30 typedef struct { // 큐 타입 element data[MAX_QUEUE_SIZE]; int front, rear; } QueueType; // 오류 함수 ..
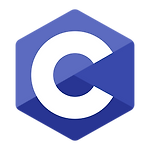
#include #include #define MAX_SIZE 5 #define ADD_F 1 #define ADD_R 2 #define REMOVE_F 3 #define REMOVE_R 4 typedef int element; typedef struct { element queue[MAX_SIZE]; int front, rear; } QueueType; void init_queue(QueueType* q){ q->front = q->rear = 0; } void error(char* msg) { fprintf(stderr, "%s\n", msg); exit(1); } int is_empty(QueueType* q) { return q->front == q->rear; } int is_full(Queue..
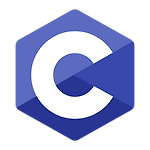
#include #include #include #define MAX_SIZE 5 /* 원형 큐 q->rear = (q->rear + 1) % MAX_SIZE; 전체 길이로 나눠서 앞에 빈 공백을 다시 재활용 따라서 is_full() 함수에 추가적인 작업이 필요함 */ typedef int element; typedef struct { element queue[MAX_SIZE]; int front, rear; } QueueType; void error(char* msg) { fprintf(stderr, "%s\n", msg); // remind this point exit(1); } void init_Queue(QueueType* q) { q->front = q->rear = 0; } int is_emp..

#include #include #define MAX_SIZE 5 /* 선형 큐 디큐로 인자를 빼는 경우 앞 공간을 활용하기가 쉽지 않다. 코드에서 디큐로 3까지 뽑았는데, 엔큐를 하면 오버플로우가 난다. 큐 배열에 데이터는 아직 존재하기 때문이다. 따라서 원형 큐로 변경하여, 빠져나간 공간을 재활용할 수 있게 만든다. */ typedef int element; typedef struct { element queue[MAX_SIZE]; int front, rear; } QueueType; void error(char* msg) { fprintf(stderr, "%s\n", msg); // remind this point exit(1); } void init_Queue(QueueType* q) { q->f..