목록ProgrammingLanguage (52)
without haste but without rest
try: from selenium import webdriver except ImportError: print("Trying to Install required module: selenium\n") os.system('python -m pip install selenium') from selenium import webdriver
순열 from itertools import permutations my_list = ['0', '1', '2', '3'] print(list(map(''.join, permutations(my_list, 2)))) 조합 from itertools import combinations my_list = ['0', '1', '2', '3'] print(list(map(''.join, combinations(my_list, 2))))

0. 개요 - 장점 1. 워킹디렉토리를 변경하거나 새로 시작해도 라이브러리를 다시 설치할 필요가 없다. 2. 텐서플로우 같은 라이브러리는 가상환경을 잡고 쓰지 않으면 구동이 안되는 경우가 있다. 하지만 이 모든게 귀찮다면 colab으로 가자... 1. 아나콘다 프롬프트에서 가상환경 생성 conda create -n env_name 아나콘다 프롬프트 환경에서 해당 명령어로 가상환경을 잡을 수 있다. conda activate env_name 위 명령어로 가상환경을 구동시킬 수 있다. conda deactivate 가상환경 종료 명령어 가상환경을 처음 잡고나면 사용하고자 하는 라이브러리는 다시 설치해줘야 한다. conda install 라이브러리 2. 파이참에서 가상환경 가져오기 env_test라는 로케이션..
import string lower = string.ascii_lowercase upper = string.ascii_uppercase total = string.ascii_letters # 대소문자 (소문자 -> 대문자 순서) # 리턴값은 string # list 씌우면 바로 리스트로 사용가능
from collections import Counter text = ['apple', 'banana', 'apple', 'orange'] c = Counter(text) // Counter to dictionary from collections import Counter text = ['apple', 'banana', 'apple', 'orange'] c = dict(Counter(text)) print(c) print(type(c)) 언젠가 요긴하게 쓰지 않을까 싶은 함수
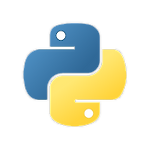
test = '"yOure,\n' token = ''.join(ch.lower() for ch in test if ch.isalnum() or ch == "'") print(token) 위 token 한줄로 텍스트 데이터 전처리시에 특수문자, 따옴표, 콤마, 마침표등 다 걸러낼 수 있다. 학교 강의에서 배운 방법인데, 스트링도 시퀀스라는 생각을 평소에 안했던 걸 반성하게 해준 코드다 .. ^^..
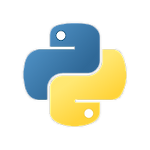
odd = True print(odd) odd = not odd print(odd) 데이터 전처리할때 써먹으면 좋다

import random # 0.0 ~ 1.0 미만 실수 반환 random.random() # a 이상 b 미만 정수 반환 random.randint(a, b) # a 이상 b 미만 정수 범위에서 interval 배수 반환 random.randrange(a, b, interval) # a 이상 b 미만 숫자 중 n개를 중복없이 반환 random.sample(range(10, 100), n)) sample_list = [1, 2, 3, 4, 5] # 랜덤 선택 # 매개변수로 받은 리스트에서 인자 n개 추출 # default 개수는 1개 random.choice(sample_list, n) # 리스트 셔플 random.shuffle(sample_list)